transformer详解
transformer详解
一、transformer简介
Transformer最早由Ashish Vaswani等人在论文<
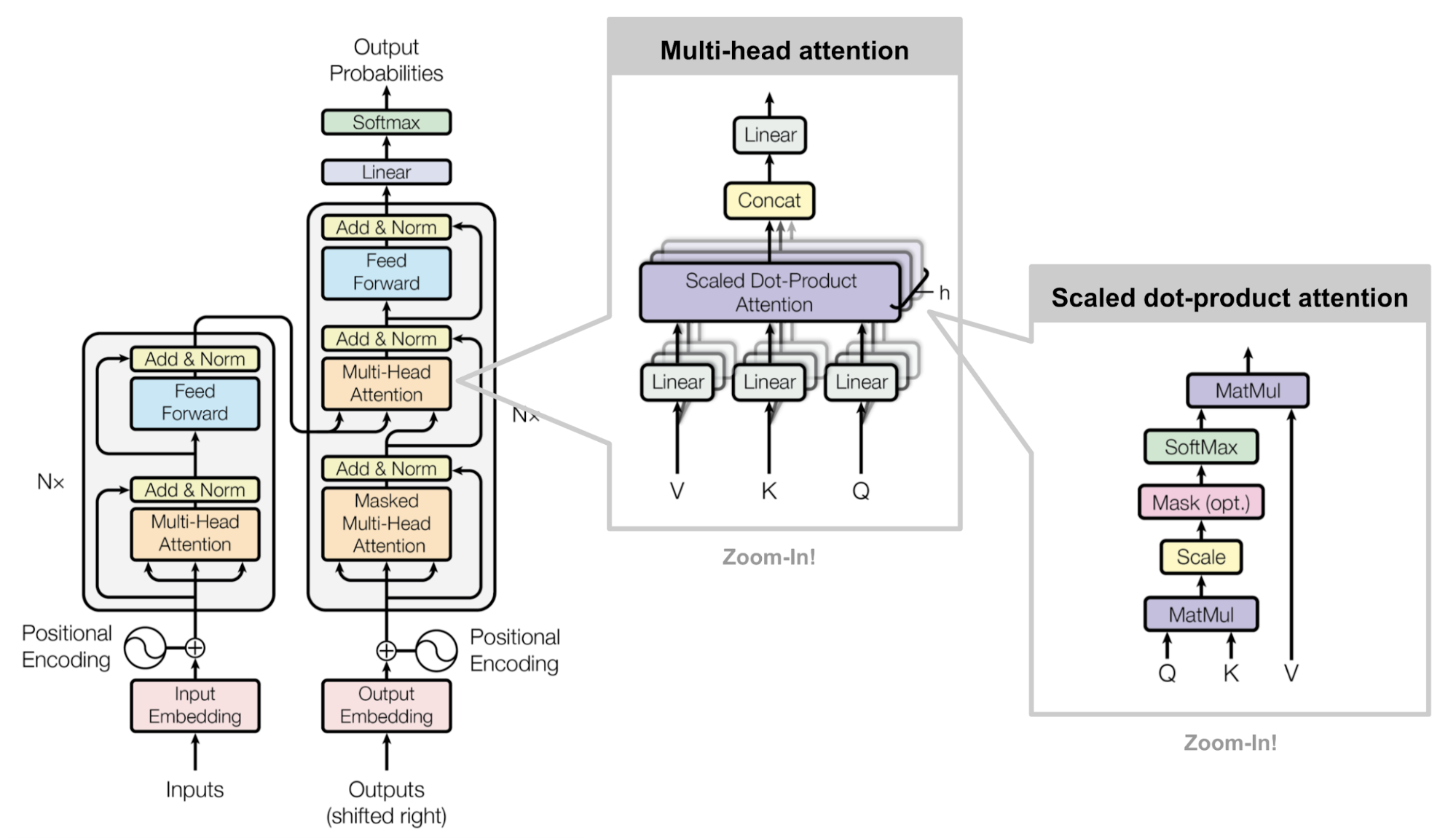
Transformer的意义体现在它的长距离依赖关系处理和并行计算,而这两点都离不开其提出的自注意力机制。
首先,Transformer引入的自注意力机制能够有效捕捉序列信息中长距离依赖关系,相比于以往的RNNs,它在处理长序列时的表现更好。
而自注意力机制的另一个特点时允许模型并行计算,无需RNN一样t步骤的计算必须依赖t-1步骤的结果,因此Transformer结构让模型的计算效率更高,加速训练和推理速度。
二、什么是自注意力机制
传统的Attention机制在一般任务的Encoder-Decoder model中,输入Source和输出Target内容是不一样的,比如对于英-中机器翻译来说,Source是英文句子,Target是对应的翻译出的中文句子,Attention机制发生在Target的元素Query和Source中的所有元素之间。简单的讲就是Attention机制中的权重的计算需要Target来参与的,即在Encoder-Decoder model中Attention权值的计算不仅需要Encoder中的隐状态而且还需要Decoder 中的隐状态。
而Self Attention顾名思义,指的不是Target和Source之间的Attention机制,而是Source内部元素之间或者Target内部元素之间发生的Attention机制,也可以理解为Target=Source这种特殊情况下的注意力计算机制。例如在Transformer中在计算权重参数时将文字向量转成对应的KQV,只需要在Source处进行对应的矩阵操作,用不到Target中的信息。
自注意力机制(Self-Attention)的核心是 Query(Q)、Key(K)、Value(V)这三个概念。这三个概念都来自于信息检索领域,其中,Query 是我们的查询,Key 是我们要匹配的目标,Value 是我们想要的结果。
注意力公式为:

对于每一个输入的句子X={x1,x2,x3,…} 每个单词x都会通过线性变换(矩阵运算)生成对应的q,k,v。 q和k的转置相乘再经过softmax。其实相当于softmax(X.XT),计算两个都由X变换而来的矩阵的内积,其实就是计算每个单词和同一句话中其他词的相关性(在注意力机制中,序列上的每个单词都会和其它所有单词做一次点积计算注意力得分,这种注意力机制中单词之间的交互是强制的不受距离影响的,所以可以解决长距离依赖问题)。经过softmax(X.XT)归一化的权重,再次乘以X变化而来的V,就相当于加权求和了,这就得到了一个经过自注意力机制后的新向量(源自引用文章[1])。
以下我们假设q=k=v,即先不加入线性变化的权重,计算流程如下:
- 首先我们计算每个单词和其转置矩阵相乘,获得X.XT
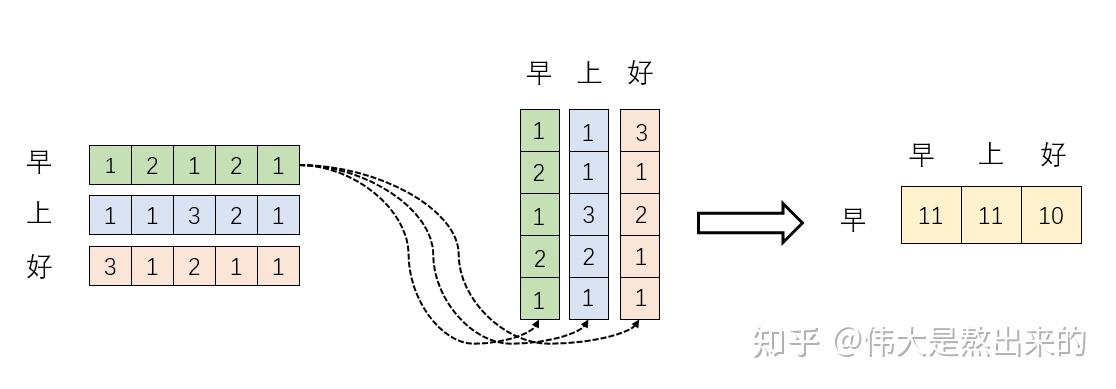
- 将其经过softmax
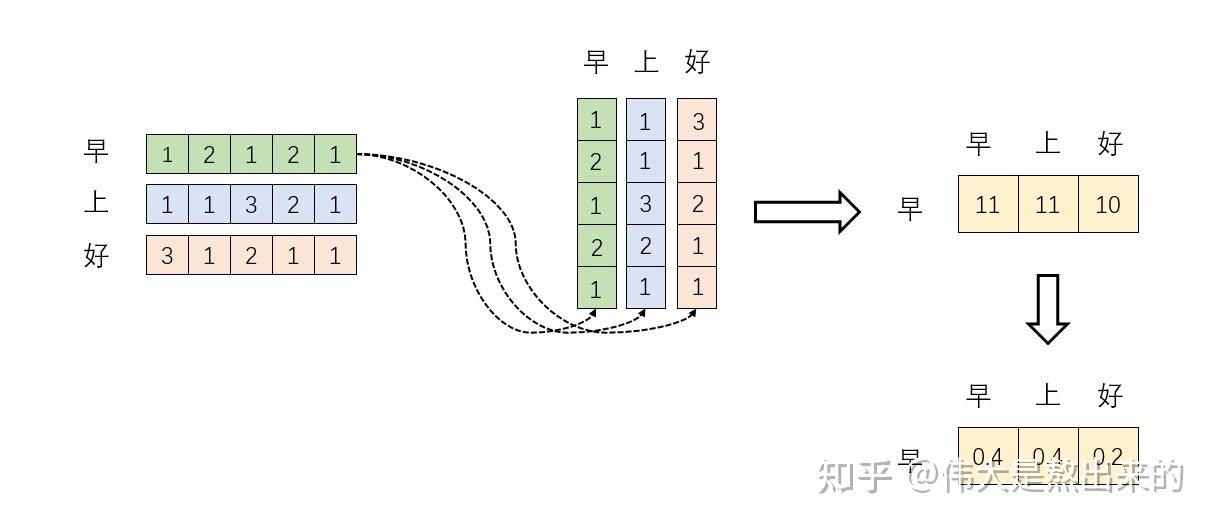
- 将注意力得分分别加到原始向量上面去,得到新的向量
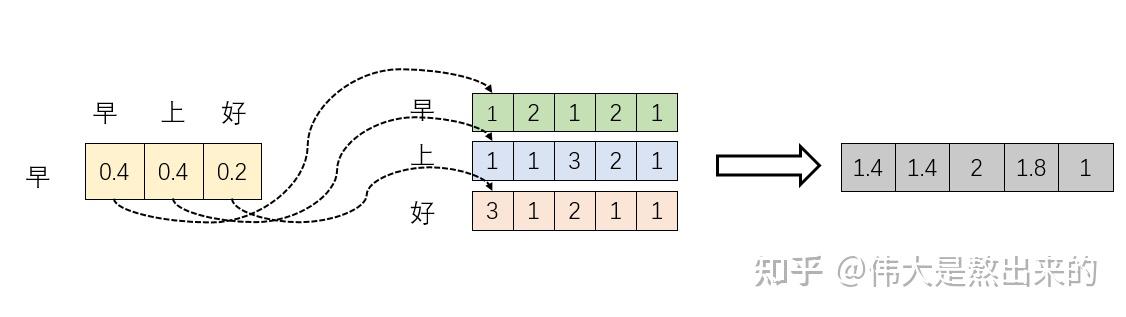
标准的self-attention计算过程如下:
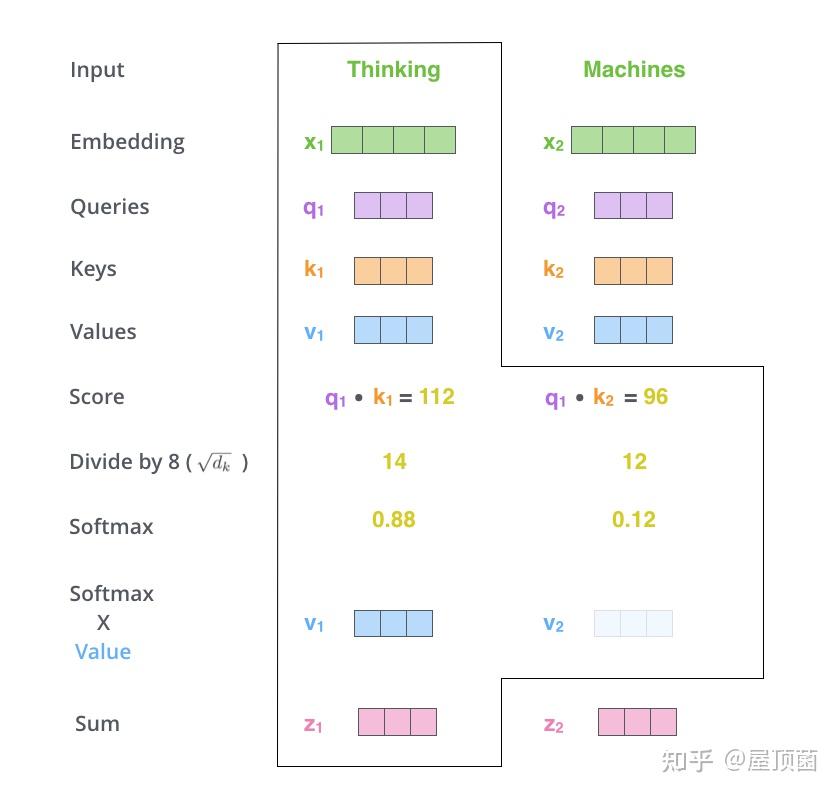
三、gpt2模型系列
1 | import transformers |
三、llama结构
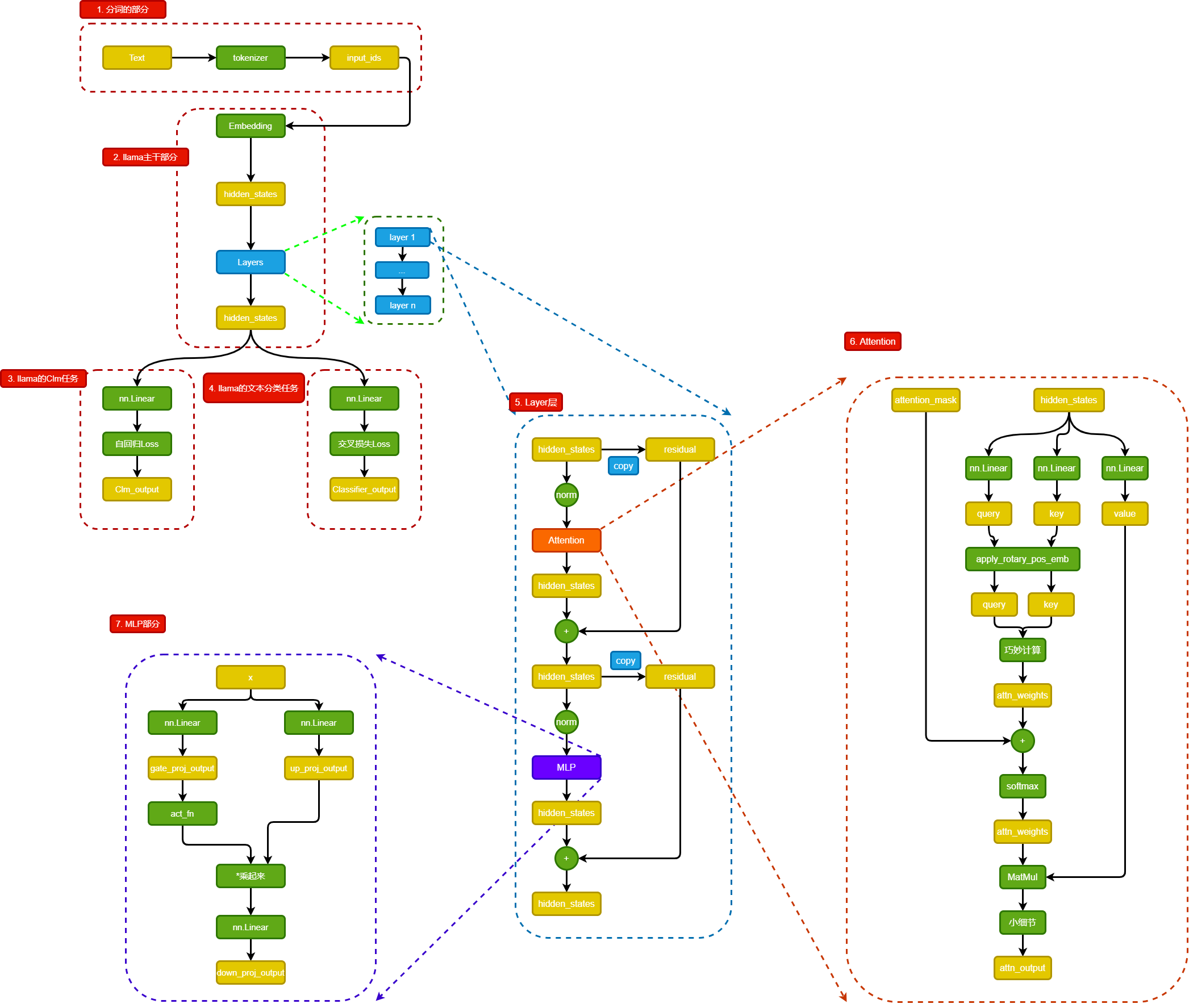
链接
- 超详细图解Self-Attention https://zhuanlan.zhihu.com/p/410776234
- 如何最简单、通俗地理解Transformer https://www.zhihu.com/question/445556653/answer/3254012065
- 熬了一晚上,我从零实现了Transformer模型,把代码讲给你听 https://zhuanlan.zhihu.com/p/411311520
- 三万字最全解析!从零实现Transformer https://zhuanlan.zhihu.com/p/648127076
- 我们从零开始用pytorch搭建Transformer模型 https://www.zhihu.com/question/445556653/answer/3145761470
- transformers可视化https://github.com/poloclub/transformer-explainer